The Problem
Google Workspace administrators can add users to a group or groups from the Admin Console user interface, up to 50 users at one user page. For larger organizations like schools or enterprises, it's inconvenient to repeat the steps to add hundreds and thousands of users in an OU to groups, e.g. 20 pages for 1000 users. While you can download the users in the OU as a CSV file, modify and upload them to a group, their CSV templates follow different formats, so it's cumbersome and error-prone to manually operate this.
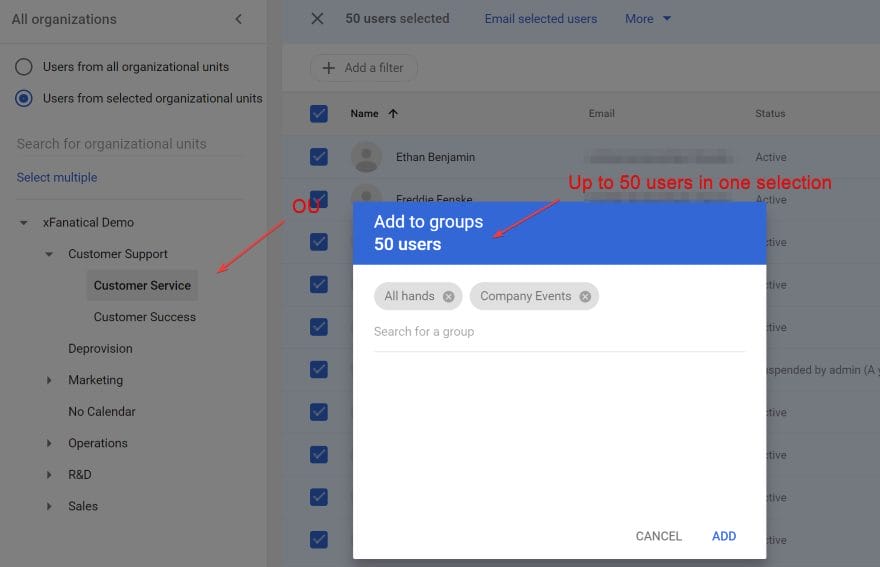
The Solution
The following open source Google Apps Script can automate the process of adding ALL users in an OU to specific groups.
Instructions
- Open https://script.google.com.
- Click New project.
- Name the script project in the title
- Copy and paste the following script to the script editor, overwriting existing
function myFunction() {}
code - Modify the
OU
andgroupEmails
variables to your organization settings - Click Services in the left navigation panel
- Select Admin SDK API
- Click Add
- Click
in the toolbar
- Click Run
- It will ask for your permissions in the first run
- After you grant the permission, the script shall run and print out the results in the Execution log.
Source code
/** * Add all users of an organizational unit (OU) to specific groups * in Google Workspace * * Usage: * Change the OU variable, in a format of /OU/SubOU/SubSubOU. The root OU is represented as / * Change the groupEmails variable, which is a list of group emails. * * © 2021 xFanatical, Inc. * @license MIT * * @since 1.0.0 proof of concept */ const OU = '/OU/SubOU/SubSubOU' const groupEmails = ['[email protected]', '[email protected]'] function addAllOUUsersToGroup() { let pageToken let page do { page = AdminDirectory.Users.list({ customer: 'my_customer', maxResults: 100, pageToken, query: `orgUnitPath='${OU}'`, }) let users = page.users if (users) { users.forEach((user) => { groupEmails.forEach((groupEmail) => { try { AdminDirectory.Members.insert({ email: user.primaryEmail, role: 'MEMBER', type: 'USER', }, groupEmail) Logger.log(`Added user [${user.primaryEmail}] to group [${groupEmail}]`) } catch (e) { Logger.log(`Failed to add user [${user.primaryEmail}] to group [${groupEmail}], error: ${e.details && e.details.message && e.details.message}`) } }) }) } else { Logger.log('No users found.') } pageToken = page.nextPageToken } while (pageToken) }
If you're interested in extending functions or automating your tasks as much as possible on Google Workspace, please check out our code-free workflow automation software Foresight. We also provide custom apps script development services to increase your productivity. Please don't hesitate to contact us. It's our passion and expertise to assist you on Google Workspace.